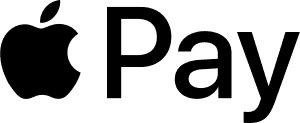
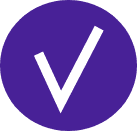
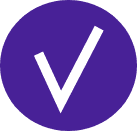
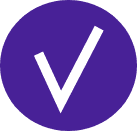
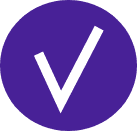
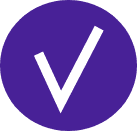
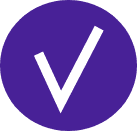
How it works?
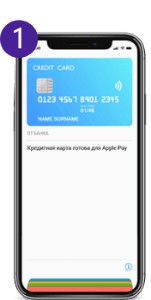
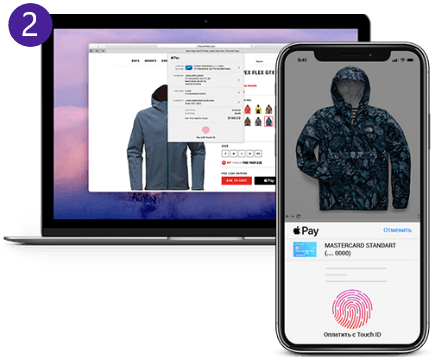
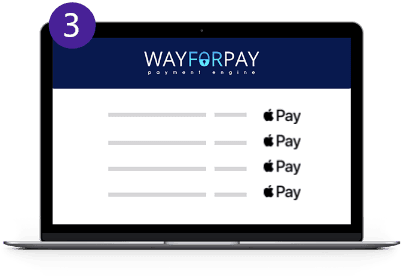
Integration
With this method, there is no need for additional integrations. The Apple Pay button will be displayed on WayForPay payment page.
If you are already registered in WayForPay, the payment method will be enabled automatically. You can manage payment methods in your Personal Account, in the "Payment Methods" menu.
With this integration a button Apple Pay can be added to your website without any additional forms. In this case, you will need to go through the connection procedure and other checks. A website or a mobile application must meet certain requirements. Your website should use HTTPS and support TLS 1.2.
Connection with Apple Pay API
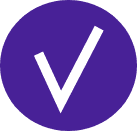
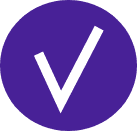
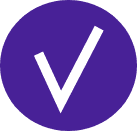
Necessary steps to use Apple Pay:
In the Apple Developer Account, go to the section «Certificates, IDs & Profiles», after «Merchant IDs» and register a new Merchant ID: in the Description field specify description; in the Identifier field must be specified the domain name of your site in the reverse order with the addition of «merchant» in the beginning (for example, the site shop.ua, the Identifier will look like - merchant.ua.shop).
Write a letter on [email protected] with your Merchant ID registered in the Apple Developer Account.
The support team will generate a certificate and send it to you with return letter. Next, in the Apple Developer console, create an Apple Pay Payment Processing Certificate and transfer it to our support team.
In the Apple Developer Account console add domains for each site where you will receive payments through Apple Pay. Sites should work under the HTTPS scheme and support the TLS 1.2 protocol.
Confirm domain ownership.
Apple Pay direct integration on website
if (window.ApplePaySession) {
var promise = ApplePaySession.canMakePaymentsWithActiveCard({YOUR_MERCHANT_ID});
promise.then(function(canMakePayments) {
if (canMakePayments) {
//the card is attached, we can start the session }
});
} else {
//impossible to pay}
document.getElementById("apple-pay-button").onclick = function(event) {
var paymentRequest = {
currencyCode: 'UAH',
countryCode: 'UA',
total: {
label: {PRODUCT_NAME},
amount: {PAYMENT_AMOUNT}
},
merchantCapabilities: ['supports3DS'],
supportedNetworks: ['masterCard', 'visa']
};
}
var session = new ApplePaySession(3, paymentRequest);
session.onvalidatemerchant = function(event) {
var promise = validateMerchant(event.validationURL);
promise.then(function(merchantSession) {
session.completeMerchantValidation(merchantSession);
});
}
function validateMerchant(validationURL) {
return new Promise(function(resolve, reject) {
var applePayPayload = {
'merchantId': {YOUR_MERCHANT_ID},
'validationURL': {YOUR_VALIDATION_URL},
'body': {
'merchantIdentifier': {YOUR_MERCHANT_ID},
'domainName': {YOUR_DOMAIN_NAME},
'displayName': {PRODUCT_NAME}
}
};
var xhr = new XMLHttpRequest();
xhr.onload = function() {
var data = JSON.parse(this.responseText);
resolve(data);
};
xhr.onerror = reject;
xhr.open('POST', merchantValidationEndpoint, true);
xhr.setRequestHeader('Content-Type', 'application/json;charset=utf-8');
xhr.send(JSON.stringify(applePayPayload));
});
}
4. Start session and process payment
session.onpaymentauthorized = function(event) {
//after receiving the token, transfer it to wayforpay
session.completePayment(ApplePaySession.STATUS_SUCCESS);
}
session.begin();
JS code example:
//in example was used jquery library//no additional scripts are required; all objects for Apple Pay payments are already in Safari
if (window.ApplePaySession) { //checking device var merchantIdentifier = 'Your Apple Merchant ID';
var promise = ApplePaySession.canMakePaymentsWithActiveCard(merchantIdentifier);
promise.then(function (canMakePayments) {
if (canMakePayments) {
$('#apple-pay').show(); //Apple Pay button }
});
}
$('#apple-pay').click(function () { //button handler var request = {
// requiredShippingContactFields: ['email'], //Uncomment if you need an e-mail. You can also request postalAddress, phone, name. countryCode: 'UA',
currencyCode: 'UAH',
supportedNetworks: ['visa', 'masterCard'],
merchantCapabilities: ['supports3DS'],
//Purpose of payment type only in Latin! total: { label: 'Test', amount: '1.00' },
}
var session = new ApplePaySession(1, request);
// event handler to create a merchant session. session.onvalidatemerchant = function (event) {
var data = {
validationUrl: event.validationURL
};
// send request to your server // for start session $.post("/ApplePay/StartSession", data).then(function (result) {
session.completeMerchantValidation(result.Model);
});
};
// payment authorization event handler session.onpaymentauthorized = function (event) {
//var email = event.payment.shippingContact.emailAddress; //if an email address was requested //var phone = event.payment.shippingContact.phoneNumber; //if phone was requested //see all options at https://developer.apple.com/reference/applepayjs/paymentcontact
var data = {
cryptogram: JSON.stringify(event.payment.token)
};
//send a request to your server, and then execute the request Charge api wayforpay.com //for payment $.post("/ApplePay/Pay", data).then(function (result) {
var status;
if (result.Success) {
status = ApplePaySession.STATUS_SUCCESS;
} else {
status = ApplePaySession.STATUS_FAILURE;
}
session.completePayment(status);
});
};
// Starting Apple Pay session session.begin();
});
Sample dataset sent by Charge wayforpay API
{
"apiVersion":1,
"transactionType":"CHARGE",
"merchantAccount":"test_merch_n1",
"merchantDomainName":"www.ggg.com",
"orderReference":"A550500763641",
"orderDate":1550500763,
"amount":1,
"currency":"UAH",
"productName":[
"dung W100F",
"Sdung d 7.0 Black"
],
"productPrice":[
21.1,
30.99
],
"productCount":[
1,
2
],
"clientFirstName":"Kgwed",
"clientLastName":"Antnd",
"clientCountry":"UKR",
"clientEmail":"[email protected]",
"clientPhone":"380612527744",
"clientIpAddress":"127.0.0.1",
"merchantSignature":"cdd678b727be03c6810f98a551a3faa0",
"merchantTransactionType":"SALE",
"merchantTransactionSecureType":"NON3DS",
"applePayString":"{"version":"EC_v1","data":"tB2ubO0fbh39SWRNB9HUEo1XjbSz65PjJl1z6VunEcIES1aLamgmdhkWRqFLcucGH5cO21magwmOXqCjip1xX83LM9okiyRXe2Rssmrb5by5OkHZeafSioqaq0n7IjDqPTu0gwtwAzpqe+0gfSnDa+M0hVWcbxe7FgXhQxLQCa7vGVbzI+d9R4Y3PdPPVIWNW9i09McNZ4Sc5xqGbHEgJ28z0K/3s2yR0O82mlxBe5SSvfTcf7rlSNBdmDq8xMYSapjv3U5YmBBAErxdaAU7bWmvEGkjONCiBJUHQzwqEhQDAnivKRTI/LiSfMs3Vv53+Vk6FHGc6DxiAClmAXBxu+Z2W+iTlzI8LYzZb4PNc6F691Ca7kfF7YIsRY+qb/rgKwsaFfHqi26cUoEV","signature":"MIAGCSqGSIb3DQEHAqCAMIACAQExDzANBglghkgBZQMEAgEFADCABgkqhkiG9w0BBwEAAKCAMIID4jCCA4igAwIBAgIIJEPyqAad9XcwCgYIKoZIzj0EAwIwejEuMCwGA1UEAwwlQXBwbGUgQXBwbGljYXRpb24gSW50ZWdyYXRpb24gQ0EgLSBHMzEmMCQGA1UECwwd92NkBh0EAgUAMAoGCCqGSM49BAMCA0gAMEUCIHKKnw+Soyq5mXQr1V62c0BXKpaHodYu9TWXEPUWPpbpAiEAkTecfW6+W5l0r0ADfzTCPq2YtbS39w01XIayqBNy8bEwggLuMIICdaADAgECAghJbS+/OpjalzAKBggqhkjOPQQDAjBnMRswGQYDVQQDDBJBcHBsZSBSb290IENBIC0gRzMxJjAkBgNVBAsMHUFwcGxlIENlcnRpZmljYXRpb24gQXV0aG9yaXR5MRMwEQYDVQQKDApBcHBsZSBJbmMuMQswCQYDVQQGEwJVUzAeFw0xNDA1MDYyMzQ2MzBaFw0yOTA1MDYyMzQ2MzBaMHoxLjAsBgNVBAMMJUFwcGxlIEFwcGxpY2F0aW9uIEludGVncmF0aW9uIENBIC0gRzMxJjAkBgNVBAsMHUFwcGxlIENlcnRpZmljYXRpb24gQXV0aG9yaXR5MRMwEQYDVQQKDApBcHBsZSBJbmMuMQswCQYDVQQGEwJVUzBZMBMGByqGSM49AgEGCCqGSM49AwEHA0IABPAXEYQZ12SF1RpeJYEHduiAou/ee65N4I38S5PhM1bVZls1riLQl3YNIk57ugj9dhfOiMt2u2ZwvsjoKYT/VEWjgfcwgfQwRgYIKwYBBQUHAQEEOjA4MDYGCCsGAQUFBzABhipodHRwOi8vb2NzcC5hcHBsZS5jb20vb2NzcDA0LWFwcGxlcm9vdGNhZzMwHQYDVR0OBBYEFCPyScRPk+TvJ+bE9ihsP6K7/S5LMA8GA1UdEwEB/wQFMAMBAf8wHwYDVR0jBBgwFoAUu7DeoVgziJqkipnevr3rr9rLJKswNwYDVR0fBDAwLjAsoCqgKIYmaHR0cDovL2NybC5hcHBsZS5jb20vYXBwbGVyb290Y2FnMy5jcmwwDgYDVR0PAQH/BAQDAgEGMBAGCiqGSIb3Y2QGAg4EAgUAMAoGCCqGSM49BAMCA2cAMGQCMDrPcoNRFpmxhvs1w1bKYr/0F+3ZD3VNoo6+8ZyBXkK3ifiY95tZn5jVQQ2PnenC/gIwMi3VRCGwowV3bF3zODuQZ/0XfCwhbZZPxnJpghJvVPh6fRuZy5sJiSFhBpkPCZIdAAAxggGMMIIBiAIBATCBhjB6MS4wLAYDVQQDDCVBcHBsZSBBcHBsaWNhdGlvbiBJbnRlZ3JhdGlvbiBDQSAtIEczMSYwJAYDVQQLDB1BcHBsZSBDZXJ0aWZpY2F0aW9uIEF1dGhvcml0eTETMBEGA1UECgwKQXBwbGUgSW5jLjELMAkGA1UEBhMCVVMCCCRD8qgGnfV3MA0GCWCGSAFlAwQCAQUAoIGVMBgGCSqGSIb3DQEJAzELBgkqhkiG9w0BBwEwHAYJKoZIhvcNAQkFMQ8XDTE5MDIxNTEwMzMyNVowKgYJKoZIhvcNAQk0MR0wGzANBglghkgBZQMEAgEFAKEKBggqhkjOPQQDAjAvBgkqhkiG9w0BCQQxIgQgDARTlPaUQwgOAWGrBpdkYCh+Yt9PR+QNrJML+rsAliowCgYIKoZIzj0EAwIERzBFAiEA9pKsQLRkwftlXnmLkTCrmFMyvi+biVCtCpizmzWoMwECIFi3+v7qhI/o/RKUbhYR6JoBUm1GswjzXhIXZiGcMq58AAAAAAAA","header":{"ephemeralPublicKey":"MFkwDQgAE88rVlkeu4xpPqMWDw59BRYO+zqqlCjcfLO3mE0/S9xhKZJfmIEcJlxtDJ1hwQ+U2L+HF7OJXL+WMsGHA==","publicKeyHash":"eUhXLM2/Z05nnAcntk0tjzFBzHgqJarnBDnovx5WMkc=","transactionId":"9845723470cc9722355763fd5b5689847842f09591855764d29322de4a319bf9"}}",
"socialUri":"socialUri",
"holdTimeout":1000000
}
Apple Pay direct integration in a mobile application
To integrate the method into the application, use the Apple SDK PassKit to get the PaymentToken and the Charge payment method in the WayForPay API for making a payment.
Payment process:




Requirements

Comission
